The Business Case for Actors and Akka.NET
From the 1980s to Present Day
Akka.NET is a .NET implementation of the actor model.
The actor model is an old technology, originating in 1973 as an approach to parallel computing at a time when it looked like the computers of the future might be constructed using thousands of small, low-powered CPUs. History didn’t turn out that way thanks to Moore’s Law; CPUs became faster and faster and modern machines were developed with a small number of very high-powered CPUs.
Despite that, the actor model is immensely popular and runs some of the world’s most important software today. Amazon’s SimpleDb, RabbitMQ, Riak, CouchBase, Goldman Sachs, Motorola, Blizzard Games, Cisco, eBay, Credit Suisse, AMN Healthcare, Bank of America, McGraw Hill Financial, and scores of other major organizations use implementations of the actor model to power mission-critical applications responsible for the world’s largest companies.
So why is the actor model so popular today? Why are so many businesses using it for mission-critical applications?
First Adopters of the Actor Model: Telecoms
The truth of the matter is, the actor model has been popular for a long time through the Erlang programming language. Erlang was the first large-scale, production usable implementation of the actor model - developed originally by Joe Armstrong as a proprietary language at Ericsson in 1986 (open sourced later) to build telephone exchanges. Today it’s used to power the GPRS, 3G, and LTE cellular networks that depend Ericsson’s products.
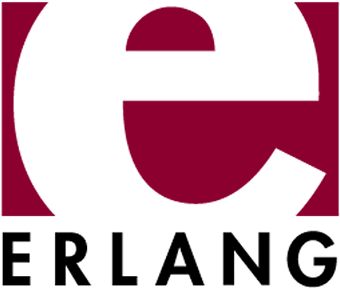
Although the actor model was originally developed as a means for running applications on types of computer hardware that never really took off, the emergence of electronic computer networks in the late 70s and early 80s gave the actor model an extremely viable commercial application: distributed and concurrent systems.
As the Internet grew and more...